Sometimes, you may want to hide the AppBar of your Flutter app when the device orientation is landscape (but still show the AppBar in portrait mode) in order to have more free space. To archive so, you can do as shown below:
Scaffold(
appBar: MediaQuery.of(context).orientation == Orientation.landscape
? null // show nothing in lanscape mode
: AppBar(
title: const Text('Kindacode.com'),
),
body: Container(/* ...*/)
);
Demo:
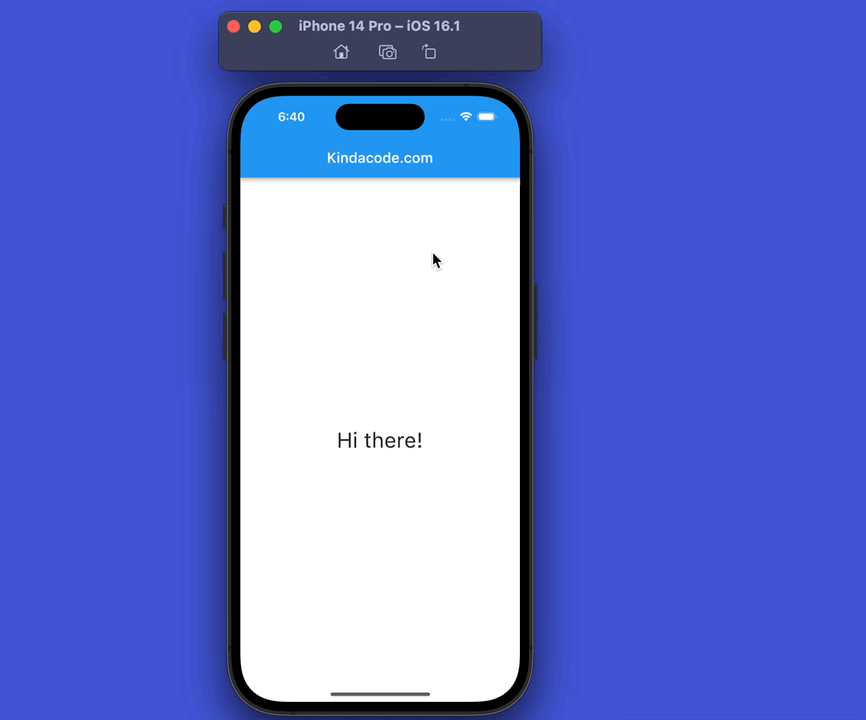
Full code in main.dart:
// main.dart
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return const MaterialApp(
// Remove the debug banner
debugShowCheckedModeBanner: false,
title: "Kindacode.com",
home: HomePage(),
);
}
}
class HomePage extends StatelessWidget {
const HomePage({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: MediaQuery.of(context).orientation == Orientation.landscape
? null // show nothing in lanscape mode
: AppBar(
title: const Text('Kindacode.com'),
),
body: Container(
alignment: Alignment.center,
child: const Text(
'Hi there!',
style: TextStyle(fontSize: 30),
),
));
}
}
That’s it. Further reading:
- Flutter: AbsorbPointer example
- Flutter LayoutBuilder Examples
- Flutter: Turn an Image into a Base64 String and Vice Versa
- Flutter: ValueListenableBuilder Example
- Flutter: ListView Pagination (Load More) example
- Flutter: Save and Retrieve Colors from Database or File
You can also take a tour around our Flutter topic page and Dart topic page to see the latest tutorials and examples.