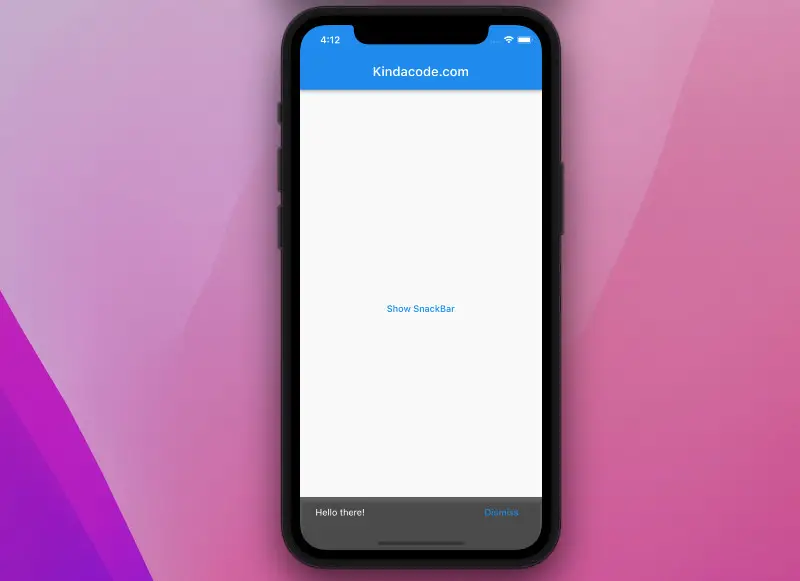
Table of Contents
TL;DR
You can hide the current SnackBar before the end of its duration by using:
ScaffoldMessenger.of(context).hideCurrentSnackBar();
This technique is useful in some cases, such as:
- The user wants to dismiss the SnackBar by tapping a button.
- A new SnackBar needs space to show up.
Complete Example
App Preview
The sample app we are going to build has a text button. When you press that text button, a snack bar that contains a Dismiss button will show up in the bottom area. If you do nothing, the snack bar will stay there for 30 seconds. However, we can manually hide it by tapping the Dismiss button.
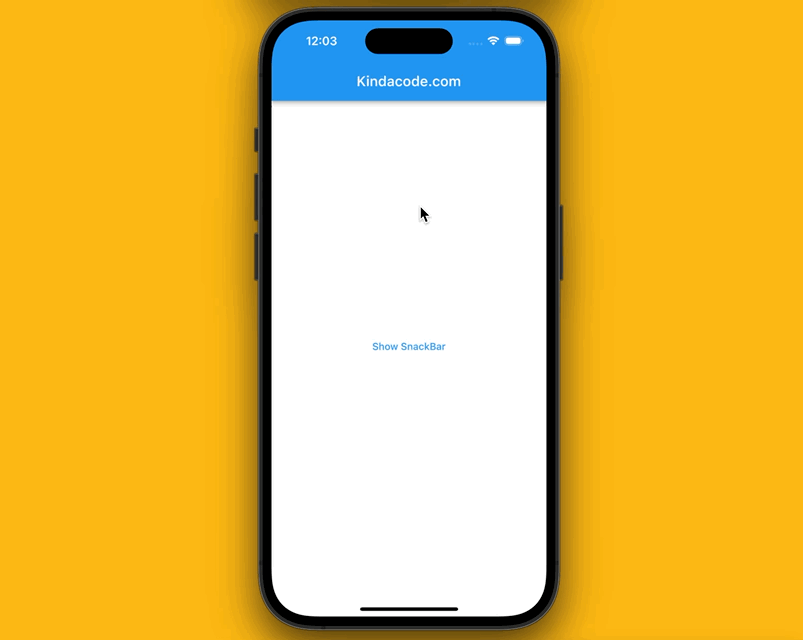
The Code
Here’s the full code, accompanied by some explanations in the comments:
// kindacode.com
// main.dart
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return MaterialApp(
// Hide the debug banner
debugShowCheckedModeBanner: false,
title: 'Kindacode.com',
theme: ThemeData.light(),
home: const HomePage(),
);
}
}
class HomePage extends StatelessWidget {
const HomePage({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Kindacode.com'),
),
body: Builder(
builder: (BuildContext ctx) {
return SafeArea(
child: Center(
child: TextButton(
child: const Text('Show SnackBar'),
onPressed: () {
// show the snackbar
ScaffoldMessenger.of(ctx).showSnackBar(SnackBar(
content: const Text('Hello there!'),
duration: const Duration(seconds: 30),
backgroundColor: Colors.black54,
action: SnackBarAction(
label: 'Dismiss',
onPressed: () {
// Hide the snackbar before its duration ends
ScaffoldMessenger.of(ctx).hideCurrentSnackBar();
},
),
));
},
),
),
);
},
));
}
}
Hope this helps. Futher reading:
- Using Chip widget in Flutter: Tutorial & Examples
- Flutter: Columns with Percentage Widths
- Flutter: Making a Dropdown Multiselect with Checkboxes
- Flutter: ExpansionPanelList and ExpansionPanelList.radio examples
- Flutter: ListTile with multiple Trailing Icon Buttons
- Flutter: Add a Search Field to an App Bar (2 Approaches)
You can also take a tour around our Flutter topic page and Dart topic page to see the latest tutorials and examples.