This article is about the heights of the AppBar (material app bar) and CupertinoNavigationBar (iOS-style app bar) in Flutter.
Height of a material AppBar
The height of an AppBar is 56 regardless of mobile phones, tablets, or on the web. However, you might not want to hardcode the number 56 wherever you have to use it. Instead, we can get the AppBar height in a more dynamic and consistent way, as shown below:
AppBar().preferredSize.height
In case you need to determine the height of both an AppBar and the status bar, use this:
Scaffold.of(context).appBarMaxHeight
Example
Screenshot:
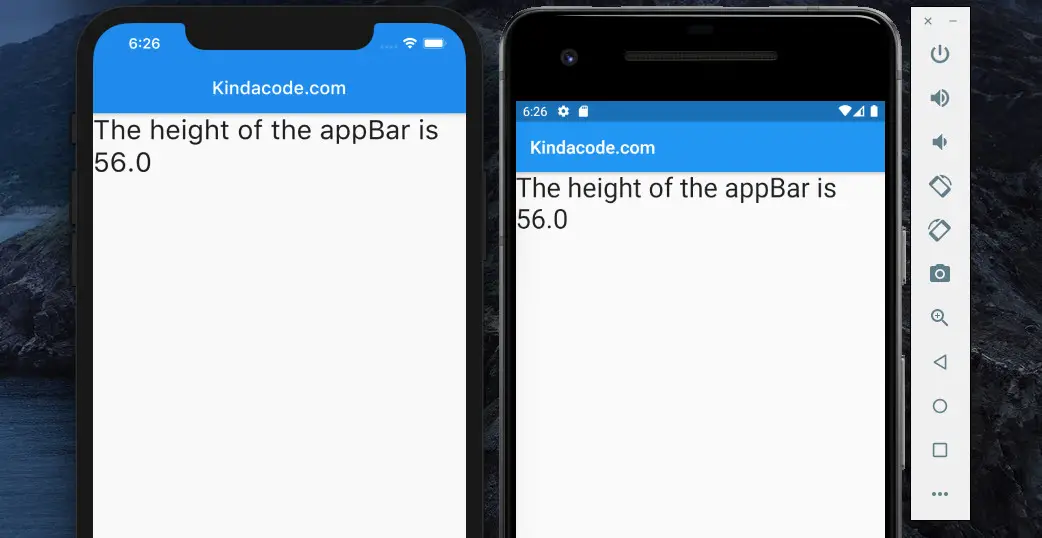
The complete code in main.dart:
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return const MaterialApp(
// Remove the debug banner
debugShowCheckedModeBanner: false,
home: MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Kindacode.com'),
),
// Display the appBar height
body: Text(
'The height of the appBar is ${AppBar().preferredSize.height}',
style: const TextStyle(fontSize: 30),
),
);
}
}
Height of a CupertinoNavigationBar
The height of a CupertinoNavigationBar (an iOS-style app bar in Flutter) is 46.0. You can retrieve it dynamically like this:
CupertinoNavigationBar().preferredSize.height
Example
Screenshot:
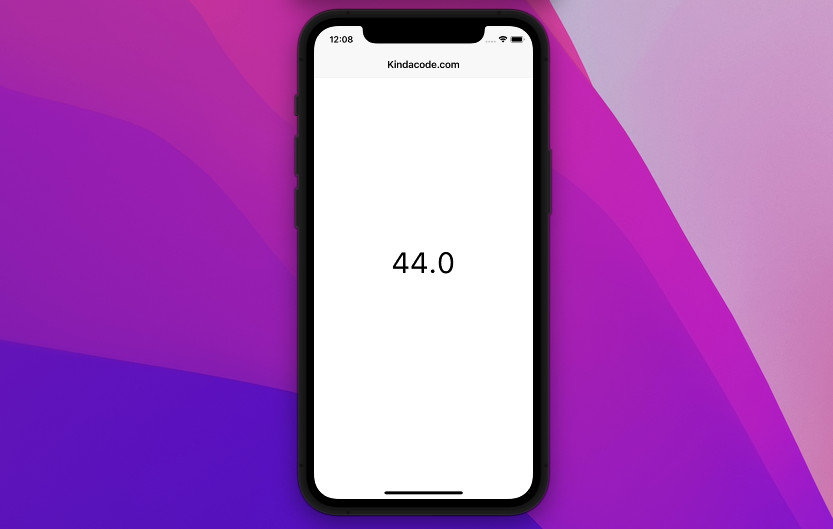
The code:
// main.dart
import 'package:flutter/cupertino.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return const CupertinoApp(
// hide the debug banner
debugShowCheckedModeBanner: false,
title: "Kindacode.com",
home: HomePage(),
);
}
}
class HomePage extends StatelessWidget {
const HomePage({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return CupertinoPageScaffold(
navigationBar: const CupertinoNavigationBar(
middle: Text('Kindacode.com'),
),
child: Center(
child: Text(
const CupertinoNavigationBar().preferredSize.height.toString(),
style: const TextStyle(fontSize: 50),
),
),
);
}
}
Conclusion
You’ve learned how to get the height of a material AppBar or a CupertinoNavigationBar in Flutter. Continue exploring more new and interesting things about this charming SDK by taking a look at the following articles:
- Flutter: SliverGrid example
- Flutter: Add a Search Field to an App Bar (2 Approaches)
- Flutter SliverAppBar Example (with Explanations)
- Flutter & Hive Database: CRUD Example
- Flutter + Firebase Storage: Upload, Retrieve, and Delete files
- Using GetX (Get) for Navigation and Routing in Flutter
You can also take a tour around our Flutter topic page and Dart topic page to see the latest tutorials and examples.