This short article is about an error that often happens when using Firebase Authentication in Flutter.
Table of Contents
The Problem
When implementing with Firebase Authentication and Flutter, you may face the MISSING_CUSTOM_TOKEN error.
The response body:
{
"error": {
"code": 400,
"message": "MISSING_CUSTOM_TOKEN",
"errors": [
{
"message": "MISSING_CUSTOM_TOKEN",
"domain": "global",
"reason": "invalid"
}
]
}
}
The common reasons cause that error are:
- Wrong API endpoint
- Invalid API Key
At the time of writing, the API endpoint looks like this:
https://identitytoolkit.googleapis.com/v1/accounts:[ACTION]?key=[API_KEY]
ACTION: signInWithCustomToken, signUp, signInWithPassword…
Note: The API endpoint structure may change over time. To get the right one, check this official guide from the Firebase website.
Solution
1. Make sure you get the right API key by going to Project settings > General > Web API Key.
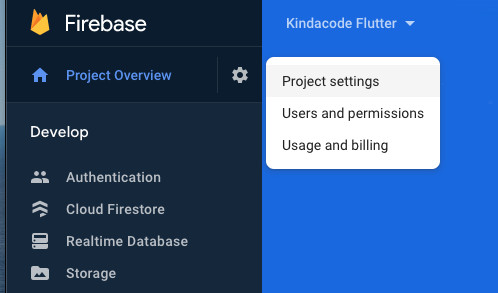
2. Review your API endpoint.
Sample Firebase Auth Code In Flutter
Install the http plugin, then implement a signUp function as follows:
import 'dart:convert';
import 'package:http/http.dart' as http;
Future<void> signUp(String email, String password) async {
final url = Uri.parse(
'https://identitytoolkit.googleapis.com/v1/accounts:signUp?key=[API_KEY]');
final response = await http.post(url, body: json.encode({
'email': email,
'password': password,
'returnSecureToken': true
}));
print(json.decode(response.body));
}
// Now call the signUp function somewhere
That’s it. Further reading:
- Flutter and Firestore Database: CRUD example
- Flutter + Firebase Storage: Upload, Retrieve, and Delete files
- Flutter: Firebase Remote Config example
- Flutter error: No Firebase App ‘[DEFAULT]’ has been created
- Flutter: Configure Firebase for iOS and Android
You can also check out our Flutter category page or Dart category page for the latest tutorials and examples.