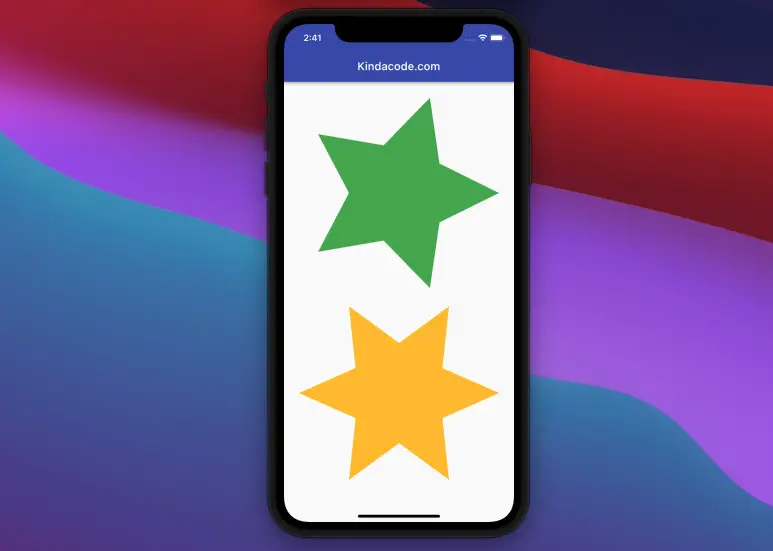
This article shows you how to draw an n-pointed star (5-pointed star, 6-pointed star, 10-pointed star, 20-pointed star, etc.) by using the CustomClipper
class in Flutter. There is no need to install any third-party packages. We will make things from scratch.
Table of Contents
What Is The Point?
1. Implement a reusable custom clipper by extending the CustomClipper
class:
// This custom clipper help us achieve n-pointed star shape
class StarClipper extends CustomClipper<Path> {
/// The number of points of the star
final int points;
StarClipper(this.points);
// Degrees to radians conversion
double _degreeToRadian(double deg) => deg * (math.pi / 180.0);
@override
Path getClip(Size size) {
Path path = Path();
double max = 2 * math.pi;
double width = size.width;
double halfWidth = width / 2;
double wingRadius = halfWidth;
double radius = halfWidth / 2;
double degreesPerStep = _degreeToRadian(360 / points);
double halfDegreesPerStep = degreesPerStep / 2;
path.moveTo(width, halfWidth);
for (double step = 0; step < max; step += degreesPerStep) {
path.lineTo(halfWidth + wingRadius * math.cos(step),
halfWidth + wingRadius * math.sin(step));
path.lineTo(halfWidth + radius * math.cos(step + halfDegreesPerStep),
halfWidth + radius * math.sin(step + halfDegreesPerStep));
}
path.close();
return path;
}
// If the new instance represents different information than the old instance, this method will return true, otherwise it should return false.
@override
bool shouldReclip(CustomClipper<Path> oldClipper) {
StarClipper starClipper = oldClipper as StarClipper;
return points != starClipper.points;
}
}
2. Now you can draw a star with ease, like this:
SizedBox(
height: 360,
width: 360,
child: ClipPath(
clipper: StarClipper(6),
child: Container(
height: 300,
color: Colors.amber,
),
),
),
The Complete Example
Preview
This example produces 4 different star shapes: a 5-pointed star, a 6-point star, a 10-pointed star, and a 20-pointed star.
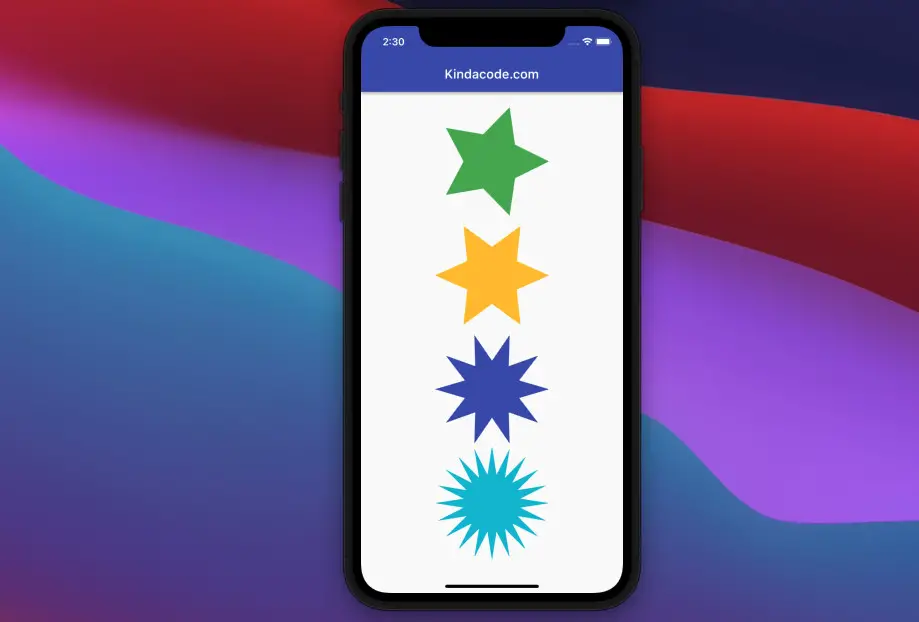
The Code
The full source code in main.dart
with explanations:
// kindacode.com
// main.dart
import 'package:flutter/material.dart';
import 'dart:math' as math;
// This custom clipper help us achieve n-pointed star shape
class StarClipper extends CustomClipper<Path> {
/// The number of points of the star
final int points;
StarClipper(this.points);
// Degrees to radians conversion
double _degreeToRadian(double deg) => deg * (math.pi / 180.0);
@override
Path getClip(Size size) {
Path path = Path();
double max = 2 * math.pi;
double width = size.width;
double halfWidth = width / 2;
double wingRadius = halfWidth;
double radius = halfWidth / 2;
double degreesPerStep = _degreeToRadian(360 / points);
double halfDegreesPerStep = degreesPerStep / 2;
path.moveTo(width, halfWidth);
for (double step = 0; step < max; step += degreesPerStep) {
path.lineTo(halfWidth + wingRadius * math.cos(step),
halfWidth + wingRadius * math.sin(step));
path.lineTo(halfWidth + radius * math.cos(step + halfDegreesPerStep),
halfWidth + radius * math.sin(step + halfDegreesPerStep));
}
path.close();
return path;
}
// If the new instance represents different information than the old instance, this method will return true, otherwise it should return false.
@override
bool shouldReclip(CustomClipper<Path> oldClipper) {
StarClipper starClipper = oldClipper as StarClipper;
return points != starClipper.points;
}
}
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return MaterialApp(
debugShowCheckedModeBanner: false,
title: 'KindaCode.com',
theme: ThemeData(
primarySwatch: Colors.indigo,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatefulWidget {
const MyHomePage({Key? key}) : super(key: key);
@override
State<MyHomePage> createState() => _MyHomePageState();
}
class _MyHomePageState extends State<MyHomePage> {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Kindacode.com'),
),
body: Padding(
padding: const EdgeInsets.all(20),
child: SingleChildScrollView(
child: Center(
child: Column(
children: [
// 5-pointed star
SizedBox(
height: 180,
width: 180,
child: ClipPath(
clipper: StarClipper(5),
child: Container(
height: 150,
color: Colors.green,
),
),
),
// 6-pointed star
SizedBox(
height: 180,
width: 180,
child: ClipPath(
clipper: StarClipper(6),
child: Container(
height: 150,
color: Colors.amber,
),
),
),
// 10-pointed star
SizedBox(
height: 180,
width: 180,
child: ClipPath(
clipper: StarClipper(10),
child: Container(
height: 150,
color: Colors.indigo,
),
),
),
// 20-pointed star
SizedBox(
height: 180,
width: 180,
child: ClipPath(
clipper: StarClipper(20),
child: Container(
height: 150,
color: Colors.cyan,
),
),
),
],
),
),
),
),
);
}
}
Conclusion
We’ve drawn custom star shapes from scratch without using any 3rd plugins. If you’d like to explore more new and exciting things in modern Flutter, take a look at the following articles:
- Flutter: Drawing Polygons using ClipPath (4 Examples)
- Adding a Border to Text in Flutter
- Flutter: How to Make Spinning Animation without Plugins
- 2 Ways to Create Flipping Card Animation in Flutter
- Flutter: Create a Button with a Loading Indicator Inside
- Flutter: Add a Search Field to an App Bar (2 Approaches)
You can also take a tour around our Flutter topic page and Dart topic page to see the latest tutorials and examples.