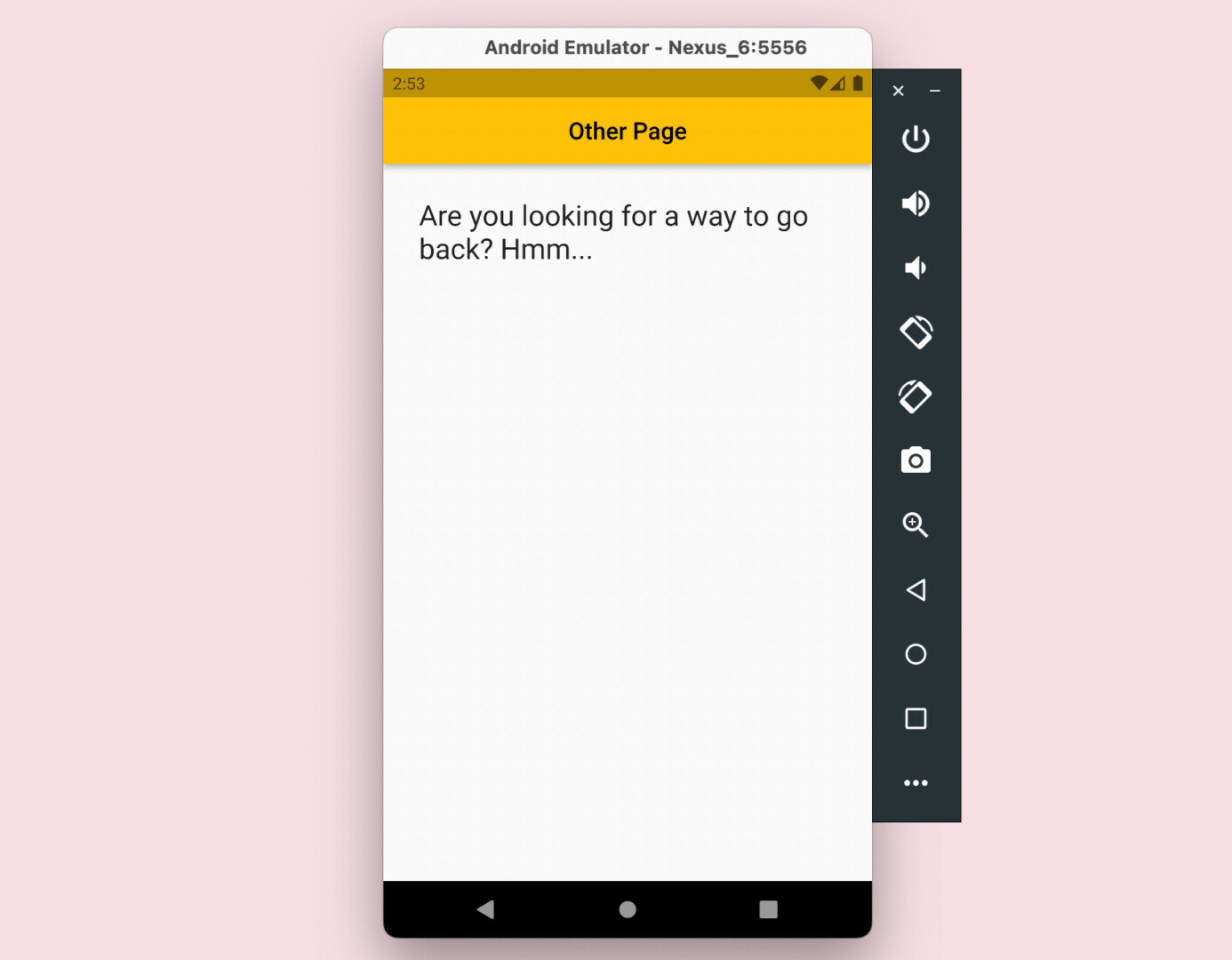
There might be cases where you want to programmatically disable the Android system back button on some specific screens in a Flutter application. This article shows you how to do it.
What Is The Point?
To prevent the Android back button from doing its job on a screen (it’s still visible), what we need to do is to wrap this screen within a WillPopScope widget and set the onWillPop parameter like this:
Widget build(BuildContext context) {
return WillPopScope(
onWillPop: () async {
/* Do something here if you want */
return false;
},
child: Scaffold(
/* ... */
),
);
}
For more clarity, please see the complete example below.
Example
App Preview
The demo app we are going to make contains 2 pages: HomePage and OtherPage. You can use the button on HomePage to navigate to OtherPage. The Android system back button is disabled so you won’t go back to HomePage when you press it. Instead, a snack bar will show up and bring a message.
Here’s how it works:
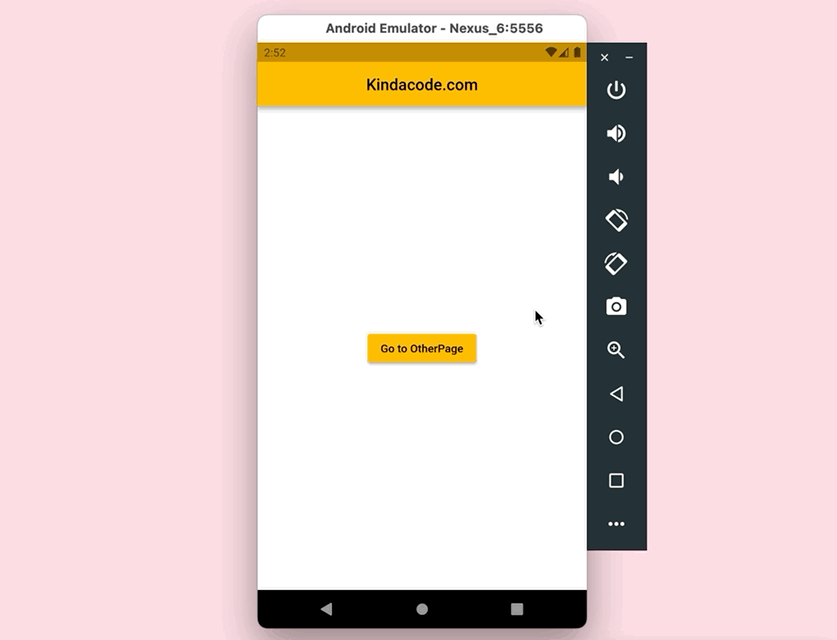
The Complete Code
// main.dart
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return MaterialApp(
// Remove the debug banner
debugShowCheckedModeBanner: false,
title: 'Kindacode.com',
theme: ThemeData(
primarySwatch: Colors.amber,
),
home: const HomePage());
}
}
// Home Page
class HomePage extends StatelessWidget {
const HomePage({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
centerTitle: true,
title: const Text('Kindacode.com'),
),
body: Center(
child: ElevatedButton(
child: const Text('Go to OtherPage'),
onPressed: () {
Navigator.of(context).push(
MaterialPageRoute(builder: (context) => const OtherPage()));
},
),
),
);
}
}
// Other Page
class OtherPage extends StatelessWidget {
const OtherPage({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return WillPopScope(
onWillPop: () async {
// show the snackbar with some text
ScaffoldMessenger.of(context).showSnackBar(const SnackBar(
content: Text('The System Back Button is Deactivated')));
return false;
},
child: Scaffold(
appBar: AppBar(
automaticallyImplyLeading: false,
centerTitle: true,
title: const Text('Other Page'),
),
body: const Padding(
padding: EdgeInsets.all(30),
child: Text(
'Are you looking for a way to go back? Hmm...',
style: TextStyle(fontSize: 24),
),
),
),
);
}
}
Conclusion
We’ve examined a full example of using the WillPopScope widget to prevent the user from using the Android system back button on a specific screen. If you’d like to learn more modern stuff in Flutter and mobile development, take a look at the following articles:
- Flutter: Customizing Status Bar Color (Android, iOS)
- Flutter: Customize the Android System Navigation Bar
- Flutter: Ask for Confirmation when Back button pressed
- Flutter & SQLite: CRUD Example
- Using GetX (Get) for Navigation and Routing in Flutter
- Flutter: 5 Ways to Add a Drop Shadow to a Widget
You can also tour around our Flutter topic page or Dart topic page for the most recent tutorials and examples.