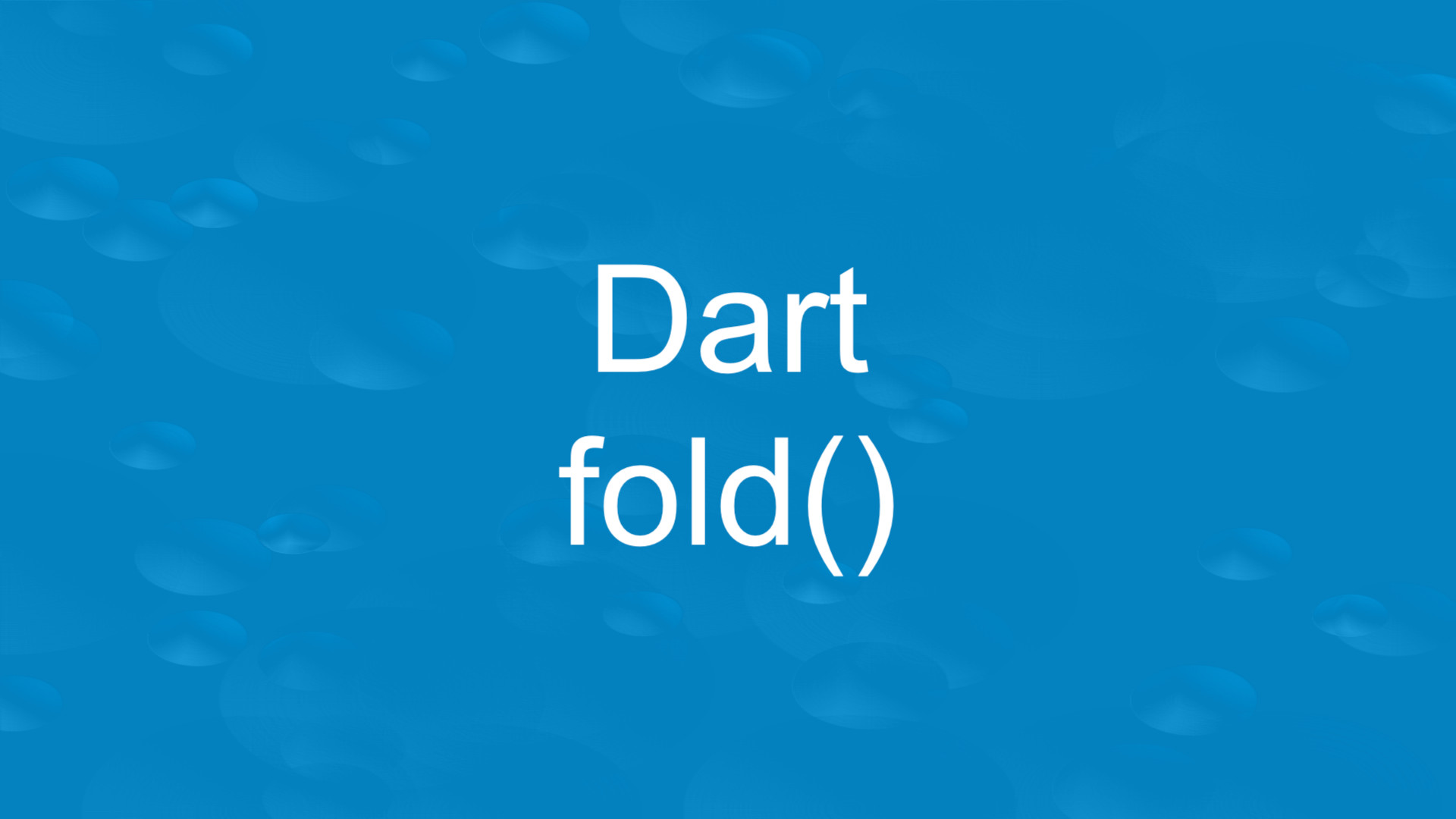
Some common use cases of the fold() method in Dart (and Flutter as well).
Calculating the Sum of a List
What this example does is simply find the sum of a given numeric list:
import 'package:flutter/foundation.dart';
void main() {
final List<int> myList = [1, 3, 5, 8, 7, 2, 11];
final int result = myList.fold(0, (sum, element) => sum + element);
if (kDebugMode) {
print(result);
}
}
Output:
37
Finding the biggest number (the maximum) in a List
The fold() method gives us an elegant way to spot the max number of a numeric list:
import 'package:flutter/foundation.dart';
void main() {
final myList = [1, 3, 5, 8, 7, 2, 11];
final int result = myList.fold(myList.first, (max, element) {
if (max < element) max = element;
return max;
});
if (kDebugMode) {
print(result);
}
}
Output:
11
Find the smallest number (the minimum) in a List
This example does the opposite thing compared to the previous one: get the min from a list of numbers.
import 'package:flutter/foundation.dart';
void main() {
final myList = [10, 3, 5, 8, 7, 2, 11];
final int result = myList.fold(myList.first, (min, element) {
if (min > element) min = element;
return min;
});
if (kDebugMode) {
print(result);
}
}
Output:
2
Wrap Up
We’ve gone over some examples of using the fold() method in Dart programs. If you’d like to explore more about Dart and Flutter development, take a look at the following articles:
- Dart & Flutter: 2 Ways to Count Words in a String
- Dart: Calculate the Sum of all Values in a Map
- Sorting Lists in Dart and Flutter (5 Examples)
- 2 ways to remove duplicate items from a list in Dart
- Flutter & Dart: Get File Name and Extension from Path/URL
- 4 ways to convert Double to Int in Flutter & Dart
You can also check out our Flutter category page, or Dart category page for the latest tutorials and examples.