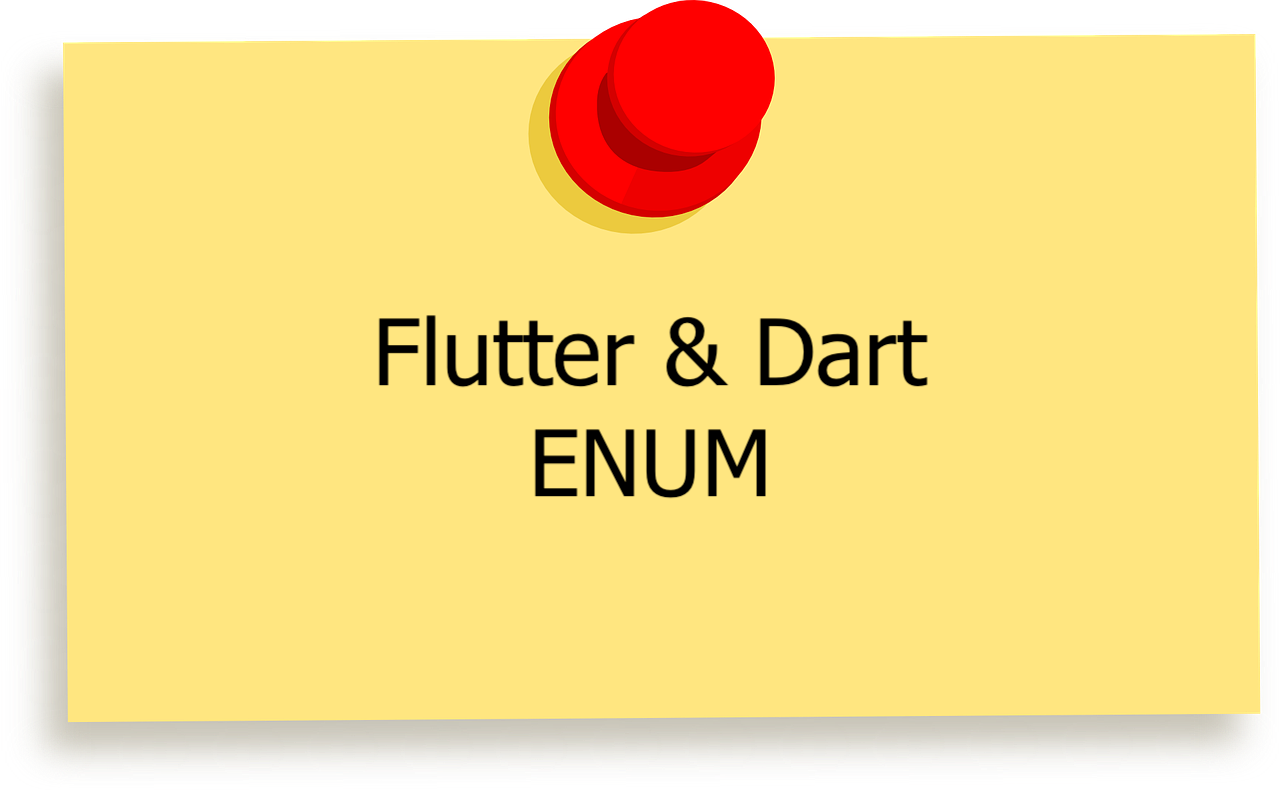
This article shows you how to use enumerations (also known as enums or enumerated types) in Dart and Flutter.
Table of Contents
Overview
An enumeration in Dart is a set of symbolic names (members) bound to unique, constant values. Within an enumeration, the members can be compared by identity, and the enumeration itself can be iterated over.
An enumeration can be declared by using the enum keyword:
enum Aniaml {dog, cat, chicken, dragon}
Every value of an enumeration has an index. The first value has an index of 0.
You can retrieve all values of an enumeration by using the values constant:
print(Aniaml.values);
// [Aniaml.dog, Aniaml.cat, Aniaml.chicken, Aniaml.dragon]
A Complete Example
The code:
// KindaCode.com
// main.dart
import 'package:flutter/foundation.dart';
// Declare enum
enum Gender { male, female }
// Make a class
class Person {
final String name;
final int age;
final Gender gender;
Person(this.name, this.age, this.gender);
}
// Create an instance
final personA = Person("John Doe", 40, Gender.male);
void main() {
if (personA.gender == Gender.male) {
debugPrint("Hello gentleman!");
} else {
debugPrint("Hi lady!");
}
}
Output:
Hello gentleman!
What’s Next?
You’ve learned the fundamentals of enumerations in Dart and Flutter. Continue exploring more new and interesting stuff by taking a look at the following articles:
- Dart: Convert Map to Query String and vice versa
- Dart: Find List Elements that Satisfy Conditions
- How to Programmatically Take Screenshots in Flutter
- Using GetX (Get) for Navigation and Routing in Flutter
- Using GetX (Get) for State Management in Flutter
- Dart: Sorting Entries of a Map by Its Values
You can also check out our Flutter category page or Dart category page for the latest tutorials and examples.