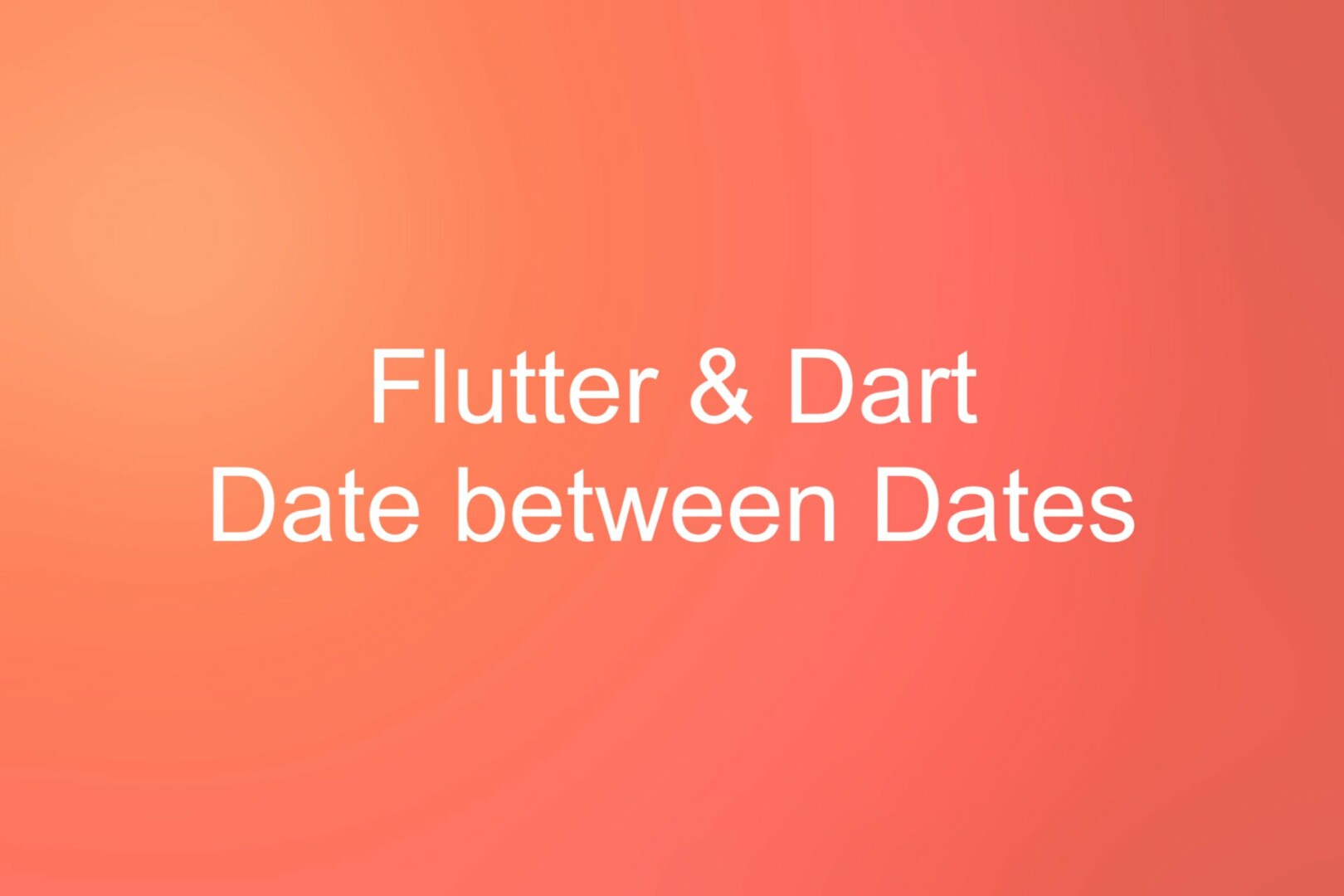
To check if a date is between 2 other ones in Flutter and Dart, you can use the isBefore() and isAfter() methods of the DateTime class.
Example
import 'package:flutter/foundation.dart';
DateTime dateA = DateTime(1900, 9, 14);
DateTime dateB = DateTime(2000, 10, 15);
DateTime dateC = DateTime(1984, 12, 20);
DateTime dateD = DateTime.now();
void main() {
if (dateA.isBefore(dateC) && dateB.isAfter(dateC)) {
debugPrint("dateC is between dateA and dateB");
} else {
debugPrint("dateC isn't between dateA and dateC");
}
if (dateA.isBefore(dateD) && dateB.isAfter(dateD)) {
debugPrint("dateD is between dateA and dateB");
} else {
debugPrint("dateD isn't between dateA and dateC");
}
}
Output:
dateC is between dateA and dateB
dateD isn't between dateA and dateC
You can find more information about the DateTime class in the official docs.
Further reading:
- Flutter: Convert UTC Time to Local Time and Vice Versa
- 2 ways to convert DateTime to time ago in Flutter
- 4 Ways to Format DateTime in Flutter
- Dart: Convert Class Instances (Objects) to Maps and Vice Versa
- How to Flatten a Nested List in Dart
- Flutter & Hive Database: CRUD Example
You can also check out our Flutter category page or Dart category page for the latest tutorials and examples.