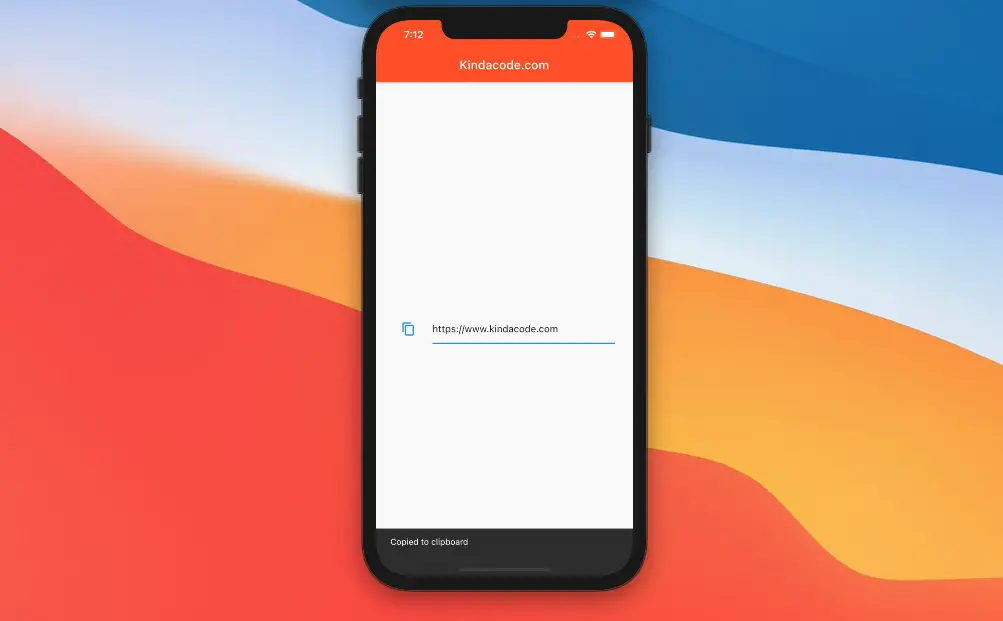
There may be situations where you want to implement Copy To Clipboard feature which allows users to copy some text (URLs, phone numbers, profile IDs…) from your app to another app (web browsers, chat apps…). The example below will show you how to do that without using any third-party plugins, thanks to the Clipboard class that comes with Flutter.
Hint: The clipboard is short-term storage used to store and transfer data within and between application programs.
Table of Contents
What is the point?
In order to use the Clipboard class, you have to import the services library:
import 'package:flutter/services.dart';
To save data to the clipboard, use the setData method (with async/await):
await Clipboard.setData(ClipboardData(text: /* your data here */));
You can also programmatically retrieve data from the clipboard with the getData method (if there is nothing, the result will be null):
final ClipboardData? _data = await Clipboard.getData('text/plain');
For more clarity, please see the complete example below.
Example
Preview
This minimal app contains a TextField that has a copy icon (actually, it’s an icon button). When this icon is pressed, the text in the TextField will be copied to the clipboard and can be pasted somewhere such as an address bar of a web browser.
In addition, a SnackBar will show up to notify the user that they have copied it.
The Code
The full source code (with explanations):
// main.dart
import 'package:flutter/material.dart';
import 'package:flutter/services.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return const MaterialApp(
debugShowCheckedModeBanner: false,
title: 'Kindacode.com',
home: HomePage(),
);
}
}
class HomePage extends StatefulWidget {
const HomePage({Key? key}) : super(key: key);
@override
State<HomePage> createState() => _HomePageState();
}
class _HomePageState extends State<HomePage> {
final TextEditingController _textController = TextEditingController();
// This function is triggered when the copy icon is pressed
Future<void> _copyToClipboard() async {
await Clipboard.setData(ClipboardData(text: _textController.text));
if (!mounted) return;
ScaffoldMessenger.of(context).showSnackBar(const SnackBar(
content: Text('Copied to clipboard'),
));
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Kindacode.com'),
backgroundColor: Colors.deepOrange,
),
body: Center(
child: Padding(
padding: const EdgeInsets.all(30),
child: TextField(
controller: _textController,
decoration: InputDecoration(
icon: IconButton(
icon: const Icon(Icons.copy),
onPressed: _copyToClipboard,
),
),
),
)));
}
}
Conclusion
We’ve examined an end-to-end example of how to implement Copy To Clipboard in Flutter. If you’d like to learn more about interesting things in Flutter, take a look at the following articles:
- Write a simple BMI Calculator with Flutter
- 4 Ways to Format DateTime in Flutter
- Flutter & Hive Database: CRUD Example
- Flutter: Scrolling to a desired Item in a ListView
- Flutter SliverList – Tutorial and Example
- 3 Ways to Create Random Colors in Flutter
You can also check out our Flutter topic page or Dart topic page for the latest tutorials and examples.