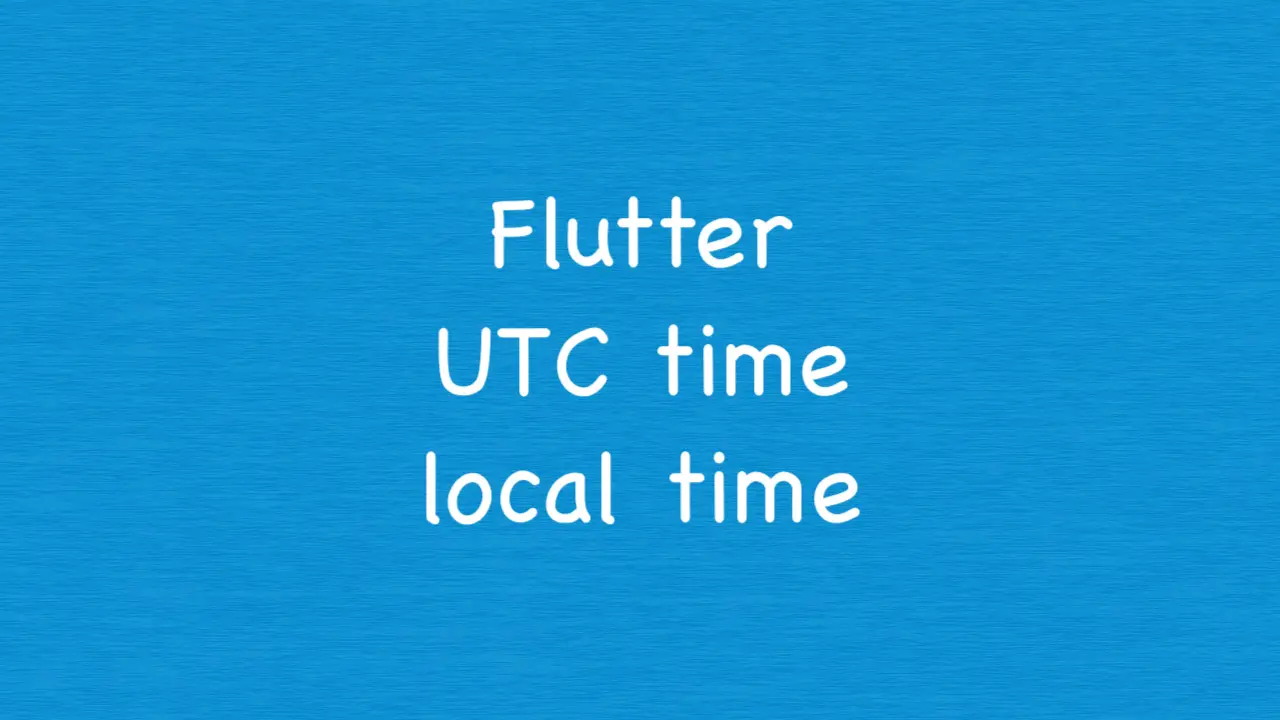
This short and straightforward article is about converting UTC time to local time and vice versa in Flutter. Without any further ado, let’s get to the point.
See Your Current Timezone
You can get your current timezone by making use of the timeZoneName property of the DateTime class:
// main.dart
void main() {
print(DateTime.now().timeZoneName);
}
You will get something like this (the result depends on your location):
-05
UTC Time to Local Time
The DateTime class has a method called toLocal() that returns a DateTime value to the local time zone.
Example:
// main.dart
void main() {
// Create a UTC DateTime object
// 2022-02-03 10:30:45
final utcTime = DateTime.utc(2023, 2, 3, 10, 30, 45);
// Get local time based on UTC time
final localTime = utcTime.toLocal();
print(localTime);
}
Output:
2023-02-03 05:30:45.000
// EST or UTC - 05:00
Local Time to UTC Time
To convert local time to UTC time, we the toUtc() method.
Example:
// main.dart
void main() {
// UTC - 5:00 (EST)
final localTime = DateTime(2023, 02, 03, 10, 22, 59);
// Convert to UTC time
final utcTime = localTime.toUtc();
print(utcTime);
}
Output:
2023-02-03 15:22:59.000Z
You can find more information about the DateTime class in the official docs.
Afterword
You’ve learned how to turn UTC time into local time and vice versa in Flutter. Continue exploring more about this awesome SDK by taking a look at the following articles:
- Dart: Convert Timestamp to DateTime and vice versa
- Ways to convert DateTime to time ago in Flutter
- Create a Custom NumPad (Number Keyboard) in Flutter
- Using GetX (Get) for State Management in Flutter
- Using Provider for State Management in Flutter
- Flutter Date Picker example
You can also take a tour around our Flutter topic page and Dart topic page to see the latest tutorials and examples.