This article walks you through 3 examples of using AnimationController in Flutter.
Simple scaling box
This example shows you how to use AnimationController in the simplest way. It creates an orange box whose size changes over time.
Note: AnimationController linearly produces the numbers from 0.0 to 1.0 during a given duration by default.
Example preview:
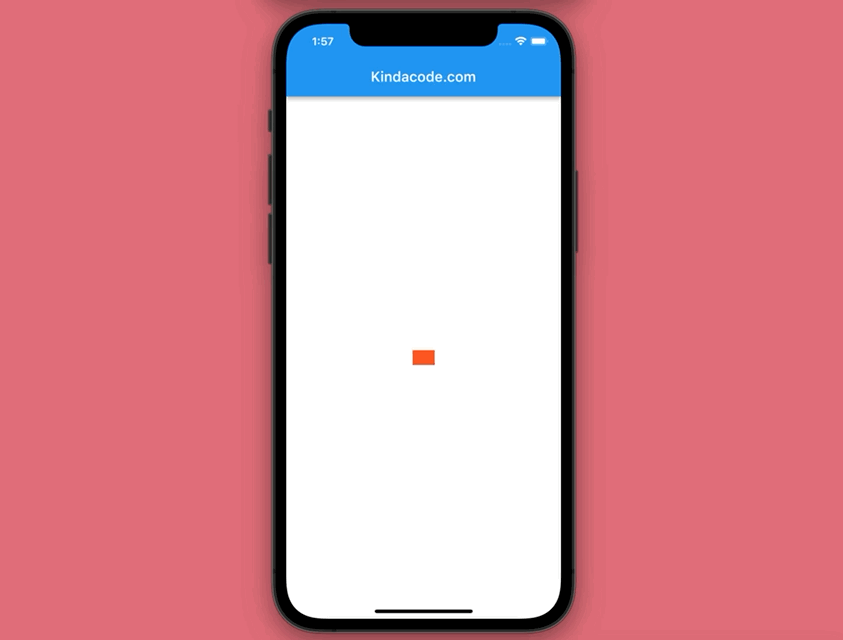
The full code:
// main.dart
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return const MaterialApp(
// Remove the debug banner
debugShowCheckedModeBanner: false,
title: 'Kindacode.com',
home: HomePage(),
);
}
}
class HomePage extends StatefulWidget {
const HomePage({Key? key}) : super(key: key);
@override
State<HomePage> createState() => _HomePageState();
}
// This class goes with TickerProviderStateMixin so that AnimationControllers can be created with "vsync: this"
class _HomePageState extends State<HomePage> with TickerProviderStateMixin {
late AnimationController _controller;
@override
void initState() {
super.initState();
_controller =
AnimationController(duration: const Duration(seconds: 3), vsync: this);
_controller.repeat(reverse: true);
}
@override
void dispose() {
_controller.dispose();
super.dispose();
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Kindacode.com'),
),
body: Center(
child: AnimatedBuilder(
animation: _controller,
builder: (BuildContext _, child) {
return Transform.scale(
scale: _controller.value,
child: child,
);
},
child: Container(
width: 300,
height: 200,
color: Colors.deepOrange,
),
),
),
);
}
}
Color Animation
Preview:
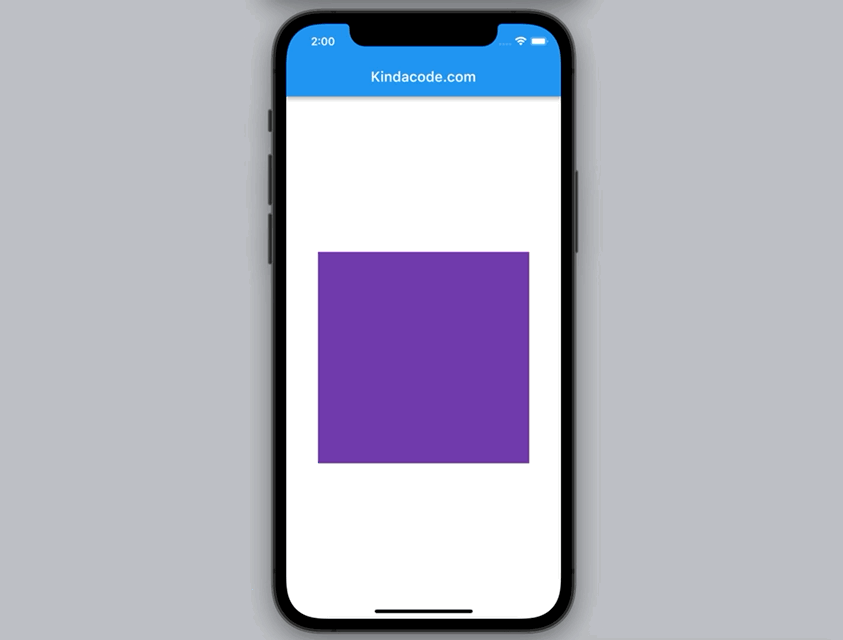
The full code:
// main.dart
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return const MaterialApp(
// Remove the debug banner
debugShowCheckedModeBanner: false,
title: 'Kindacode.com',
home: HomePage(),
);
}
}
class HomePage extends StatefulWidget {
const HomePage({Key? key}) : super(key: key);
@override
State<HomePage> createState() => _HomePageState();
}
// This class goes with TickerProviderStateMixin so that AnimationControllers can be created with "vsync: this"
class _HomePageState extends State<HomePage> with TickerProviderStateMixin {
late AnimationController _controller;
late Animation<Color?> _colorTween;
@override
void initState() {
super.initState();
_controller =
AnimationController(duration: const Duration(seconds: 3), vsync: this);
_controller.repeat(reverse: true);
_colorTween = ColorTween(begin: Colors.deepOrange, end: Colors.deepPurple)
.animate(_controller);
}
@override
void dispose() {
_controller.dispose();
super.dispose();
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Kindacode.com'),
),
body: Center(
child: AnimatedBuilder(
animation: _colorTween,
builder: (BuildContext _, Widget? __) {
return Container(
width: 300,
height: 300,
color: _colorTween.value,
);
},
),
),
);
}
}
A little bit more complicated
This example creates a box whose shape, color, and border change over time.
Preview:
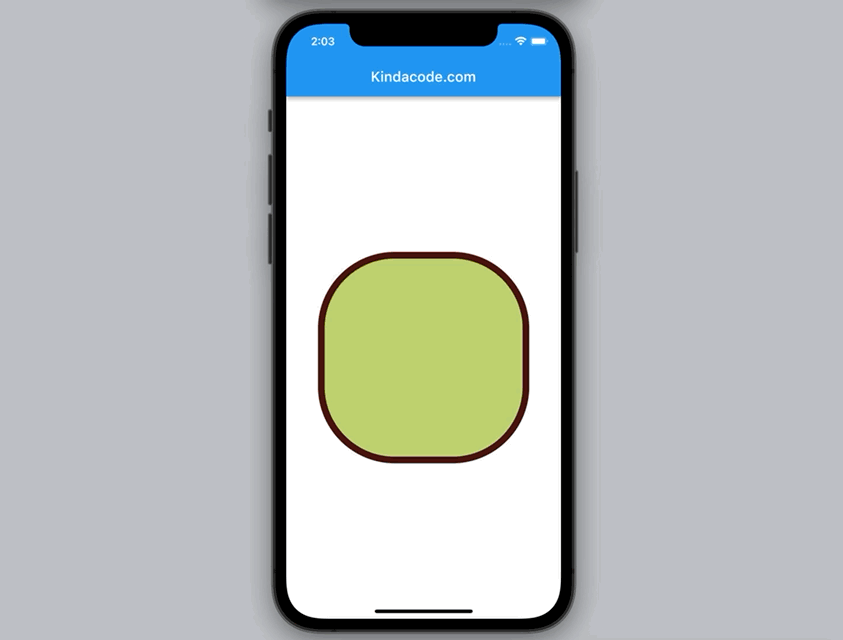
The code:
// main.dart
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return const MaterialApp(
// Remove the debug banner
debugShowCheckedModeBanner: false,
title: 'Kindacode.com',
home: HomePage(),
);
}
}
class HomePage extends StatefulWidget {
const HomePage({Key? key}) : super(key: key);
@override
State<HomePage> createState() => _HomePageState();
}
// This class goes with TickerProviderStateMixin so that AnimationControllers can be created with "vsync: this"
class _HomePageState extends State<HomePage> with TickerProviderStateMixin {
late AnimationController _controller;
late Animation<Decoration> _decorationTween;
@override
void initState() {
super.initState();
_controller =
AnimationController(duration: const Duration(seconds: 5), vsync: this);
_controller.repeat(reverse: true);
_decorationTween = DecorationTween(
begin: BoxDecoration(
color: Colors.blue,
border: Border.all(width: 30, color: Colors.red),
borderRadius: BorderRadius.circular(10)),
end: BoxDecoration(
color: Colors.yellow,
border: Border.all(width: 1, color: Colors.black),
borderRadius: BorderRadius.circular(150)))
.animate(_controller);
}
void dispose() {
_controller.dispose();
super.dispose();
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Kindacode.com'),
),
body: Center(
child: DecoratedBoxTransition(
decoration: _decorationTween,
child: const SizedBox(
width: 300,
height: 300,
)),
),
);
}
}
Wrapping Up
We’ve examined more than one example of creating animations with AnimationController in Flutter. From now on, you can make more complex and advanced effects without using any third-party packages. If you’d like to explore more new and interesting things in Flutter, take a look at the following articles:
- Flutter: ColorTween Example
- Adding and Customizing a Scrollbar in Flutter
- Flutter AnimatedList – Tutorial and Examples
- Flutter Transform examples – Making fancy effects
- Flutter: How to Make Spinning Animation without Plugins
- 2 Ways to Create Flipping Card Animation in Flutter
- Best Plugins to Easily Create Animations in Flutter
You can also take a tour around our Flutter topic page and Dart topic page to see the latest tutorials and examples.