This article shows you how to add a custom character counter to a TextField (you can also use TextFormField as well) in Flutter.
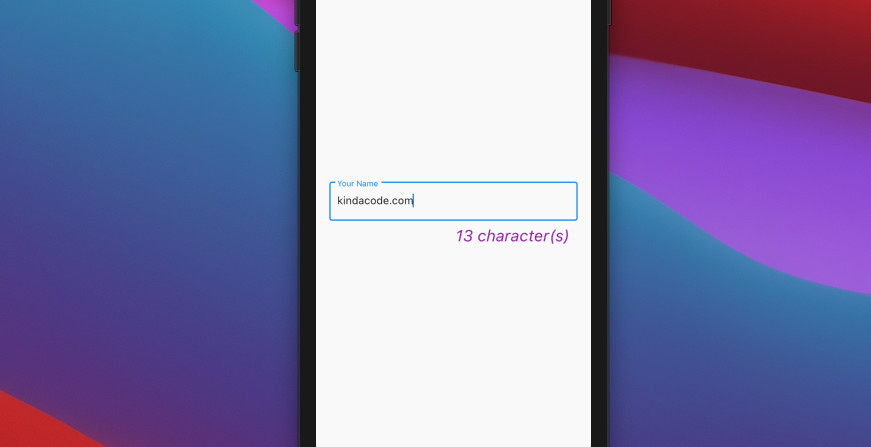
Table of Contents
Overview
Here’s the main points:
1. We will display the number of characters entered (including the spaces between words) using the counterText property of the InputDecoration class. The counter text will show up in the bottom right of the text field. You can style this thing with the counterStyle option.
2. To make the counter update when the user enters or deletes a character, we’ll have to call setState() every time the onChanged function is invoked, like this:
TextField(
onChanged: (value) {
setState(() {
/* Update the counter */
});
},
/* Other code */
)
For more clarity, see the example below.
The Complete Example
Preview
In this example, we’ll create a simple app that contains a text field in the center of the screen. The number of characters entered in the text field changes as soon as the user types something.
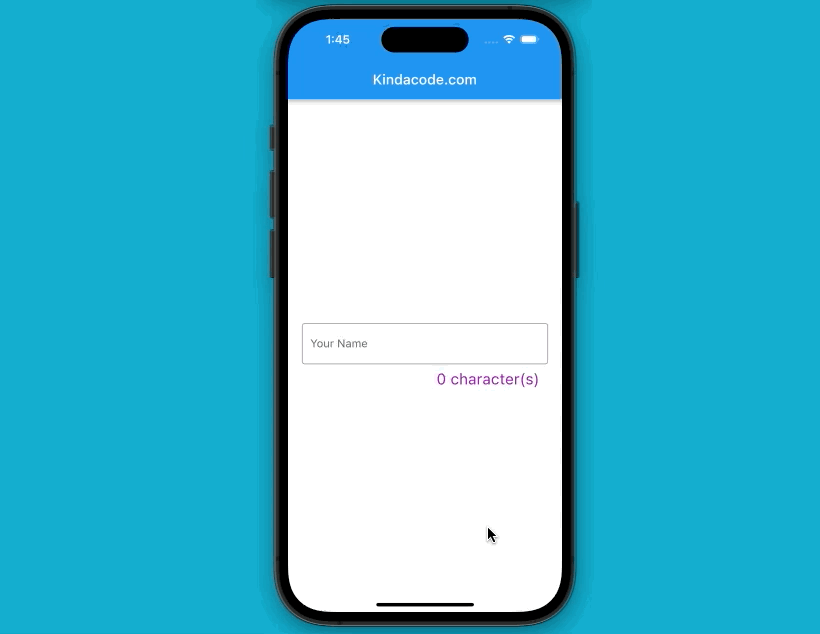
The code
Here’s the full source code in main.dart:
// KindaCode.com
// main.dart
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return const MaterialApp(
// Remove the debug banner
debugShowCheckedModeBanner: false,
title: 'Kindacode.com',
home: HomePage(),
);
}
}
class HomePage extends StatefulWidget {
const HomePage({Key? key}) : super(key: key);
@override
State<HomePage> createState() => _HomePageState();
}
class _HomePageState extends State<HomePage> {
// This variable holds the text typeed by the user
String _enteredText = '';
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Kindacode.com'),
),
body: Padding(
padding: const EdgeInsets.all(20),
child: Center(
child: TextField(
onChanged: (value) {
setState(() {
_enteredText = value;
});
},
decoration: InputDecoration(
labelText: 'Your Name',
border: const OutlineInputBorder(),
// Display the number of entered characters
counterText: '${_enteredText.length.toString()} character(s)',
// style counter text
counterStyle:
const TextStyle(fontSize: 22, color: Colors.purple)),
),
),
),
);
}
}
Note: You can replace TextField with TextFormField, and the code above will give the same result.
Conclusion
You’ve learned how to add a letter counter to a TextField in Flutter. Continue learning more interesting stuff by having a look at the following articles:
- Flutter StreamBuilder examples
- Flutter and Firestore Database: CRUD example
- Customize Borders of TextField/TextFormField in Flutter
- How to set width, height, and padding of TextField in Flutter
- How to check your Flutter and Dart versions
- Using AnimatedIcon in Flutter
You can also check out our Flutter topic page or Dart topic page for the latest tutorials and examples.