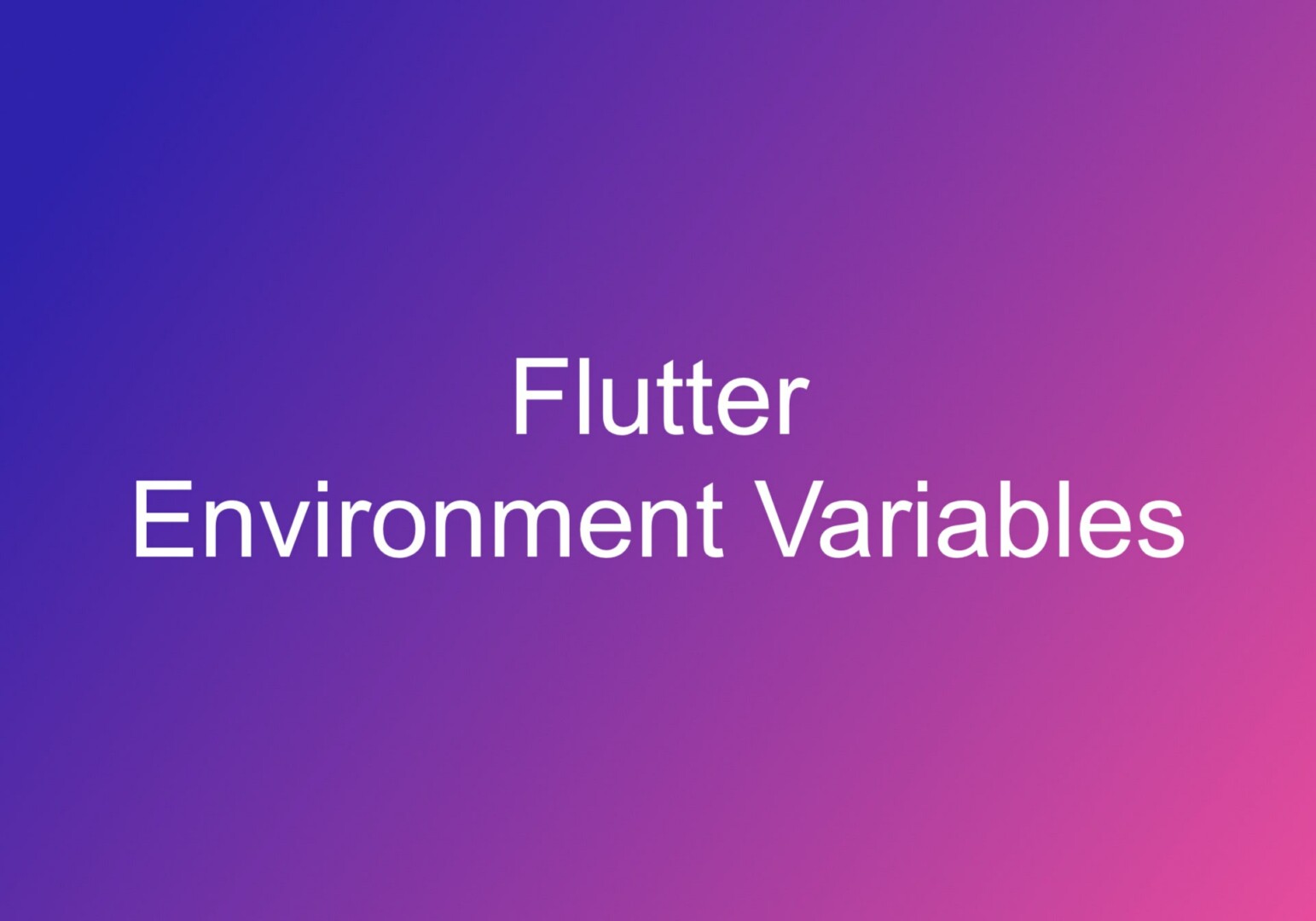
This article shows you a couple of different ways to use different configurations for development and production in Flutter.
Using the dart.vm.product environment flag
You can determine whether your app is currently running in production or development like so:
bool isProd = const bool.fromEnvironment('dart.vm.product');
Example
Let’s say we have an app that works with a backend. When developing and debugging the app, we want it to make HTTP requests with a testing server on localhost. When the app is released to the world, we want it to communicate with our production REST API.
In the lib folder, create a new file named config.dart. The file structure will look like this:
.
├── config.dart
└── main.dart
Here’s the code in config.dart:
// config.dart
String get apiHost {
bool isProd = const bool.fromEnvironment('dart.vm.product');
if (isProd) {
return 'https://www.kindacode.com/api/some-path';
// replace with your production API endpoint
}
return "https://localhost/api/some-path";
// replace with your own development API endpoint
}
From now on you can import apiHost to use wherever you need to send GET or POST requests:
// import config file
import './config.dart' show apiHost;
void main() {
print(apiHost);
}
This approach is neat and convenient.
Adding ENV variables via the command line
If you don’t want to put your settings in your Dart code, you can add environment variables when running flutter run and flutter build commands.
Example
Development:
flutter run --dart-define=API_HOST=http://localhost/api --dart-define=API_KEY=123
Production:
flutter build --dart-define=API_HOST=https://www.kindacode.com/api --dart-define=API_KEY=secret
You can retrieve your environment variables in your Dart code like this:
// you can set default values for your variables
// the will be used if you forget to add values when executing commands
const apiHost = String.fromEnvironment("API_HOST", defaultValue: "http://localhost/api");
const apiKey = String.fromEnvironment("API_KEY", defaultValue: "123");
Conclusion
We’ve explored more than one approach to apply different settings for an app based on the environment it’s running in. If you’d like to learn more new and interesting things about Flutter, take a look at the following articles:
- Using NavigationRail and BottomNavigationBar in Flutter
- Best Libraries for Making HTTP Requests in Flutter
- Using GetX (Get) for Navigation and Routing in Flutter
- How to implement Star Rating in Flutter
- How to save network images to the device in Flutter
You can also take a tour around our Flutter topic page and Dart topic page to see the latest tutorials and examples.