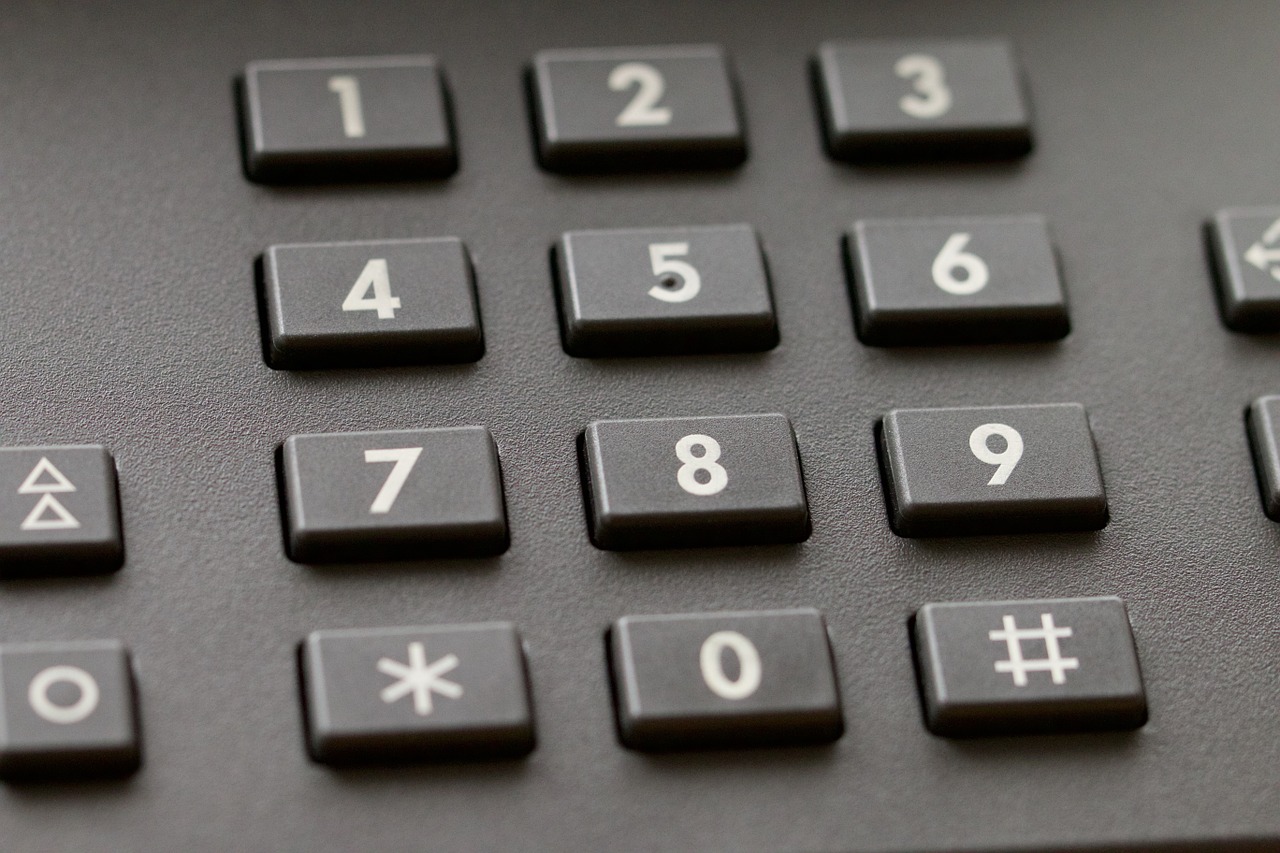
Validating phone numbers is a common task when making Flutter applications. Before sending an SMS with a verification code (that usually costs real money), we should implement some basic checks.
In this article, we’ll use a regular expression pattern to validate the United States and Canada phone numbers that match the following formats:
(123) 456 789
321-123-4567
+01 (123) 456 7890
+1 123 456 7890
123 456 7890
Table of Contents
The phone regex pattern
Here’s the phone regex pattern used in our Dart code:
^(\+0?1\s)?((\d{3})|(\(\d{3}\)))?(\s|-)\d{3}(\s|-)\d{4}$
Explanation:
- ^(\+0?1\s)?: Check international code
- ((\d{3})|(\(\d{3}\)))?: Check area code
- (\s|-): Check separator (whitespace, dash)
- \d{3}: Check the 3 middle digits
- (\s|-): Check separator (whitespace, dash)
- \d{4}$: Check the last 4 digits
Example
The code:
// Define a function that is reusable
void _check(String input) {
final RegExp phone =
RegExp(r'^(\+0?1\s)?((\d{3})|(\(\d{3}\)))?(\s|-)\d{3}(\s|-)\d{4}$');
if (phone.hasMatch(input)) {
print('$input is valid');
} else {
print('$input is not valid');
}
}
// Test it
void main() {
_check('+01 (123) 456 7890');
_check('123 456 7890');
_check('123 456-7890');
_check('(123) 456-7890');
_check('123-456-7890');
_check('123 4432 234');
}
Output:
+01 (123) 456 7890 is valid
123 456 7890 is valid
123 456-7890 is valid
(123) 456-7890 is valid
123-456-7890 is valid
123 4432 234 is not valid
Final Words
Our solution is good enough for client-side checking, but it isn’t perfect. Writing a perfect regex pattern that works in any situation is super challenging, even with common stuff like phone numbers or emails… It will take you a lot of time and effort and maybe reduce the application performance.
Continue learning more new and fascinating things about modern Flutter by taking a look at the following articles:
- Dart regular expressions to check people’s names
- Flutter & Dart: Regular Expression Examples
- Flutter form validation example
- Flutter: Making Beautiful Chat Bubbles (2 Approaches)
- Flutter: Creating OTP/PIN Input Fields (2 approaches)
- Flutter StreamBuilder examples (null safety)
You can also take a tour around our Flutter topic page and Dart topic page to see the latest tutorials and examples.