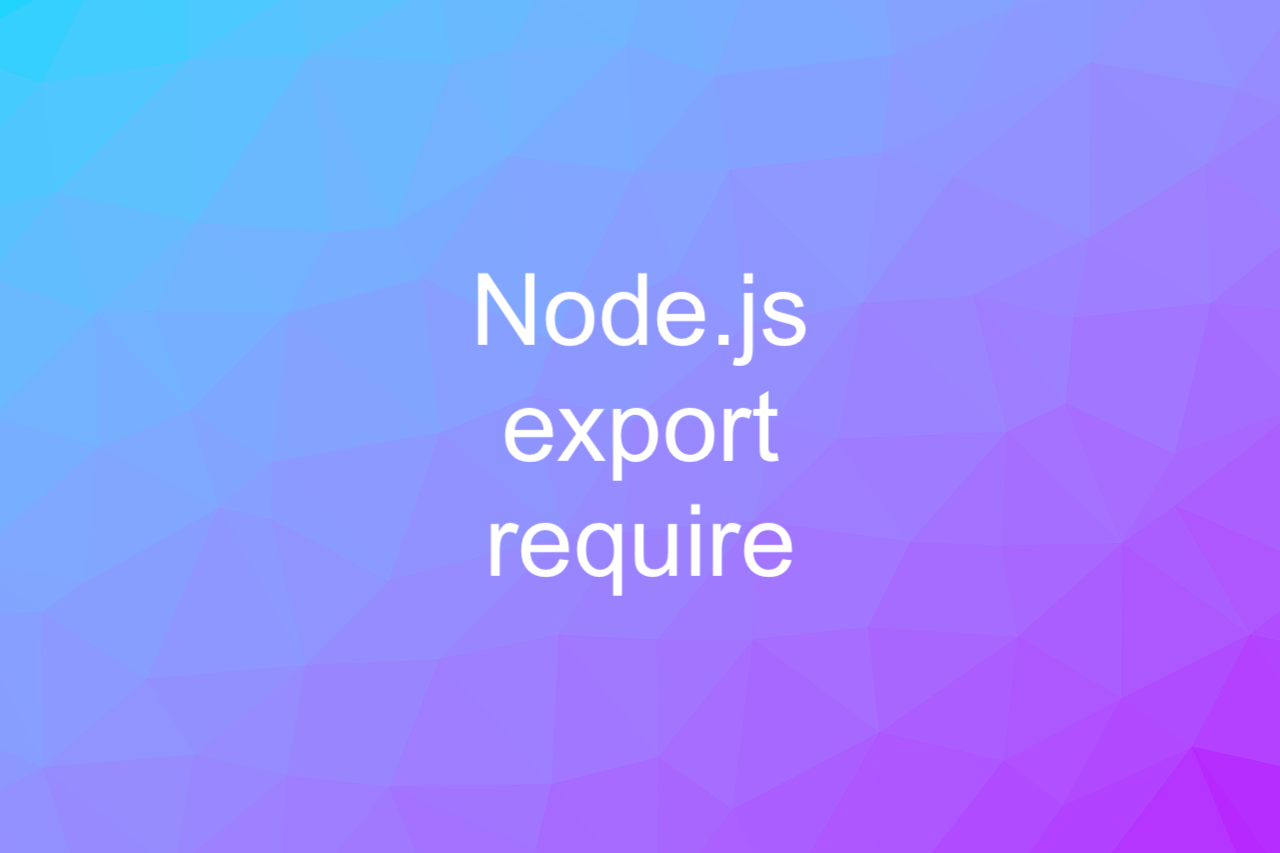
A few easy-to-understand examples that will help you understand “exports” and “require” in Node.js better.
Example 1
Exports:
// your-file.js
const firstMethod = () => {
// do something
}
const secondMethod = () => {
// do something
}
const text = 'Some text';
module.exports.first = firstMethod;
module.exports.second = secondMethod;
module.exports.text = text;
// All the names are depend on your own
Require (file index.js)
const { first, second, text } = require("./your-file");
// Execution
first();
second();
console.log(text);
Examples 2
Export (call it your-file.js or whatever you like):
// your-file.js
const firstMethod = () => {
// do something
}
const secondMethod = () => {
// do something
}
const text = 'Some text';
module.exports = {
first: firstMethod,
second: secondMethod,
text: text
}
Require:
const x = require("./your-file");
// Execution
x.first();
x.second();
console.log(x.text);
Further reading:
- 2 Ways to Set Default Time Zone in Node.js
- Node.js: How to Compress a File using Gzip format
- 4 Ways to Read JSON Files in Node.js
- Node.js: Using __dirname and __filename with ES Modules
You can also check out our Node.js category page for the latest tutorials and examples.