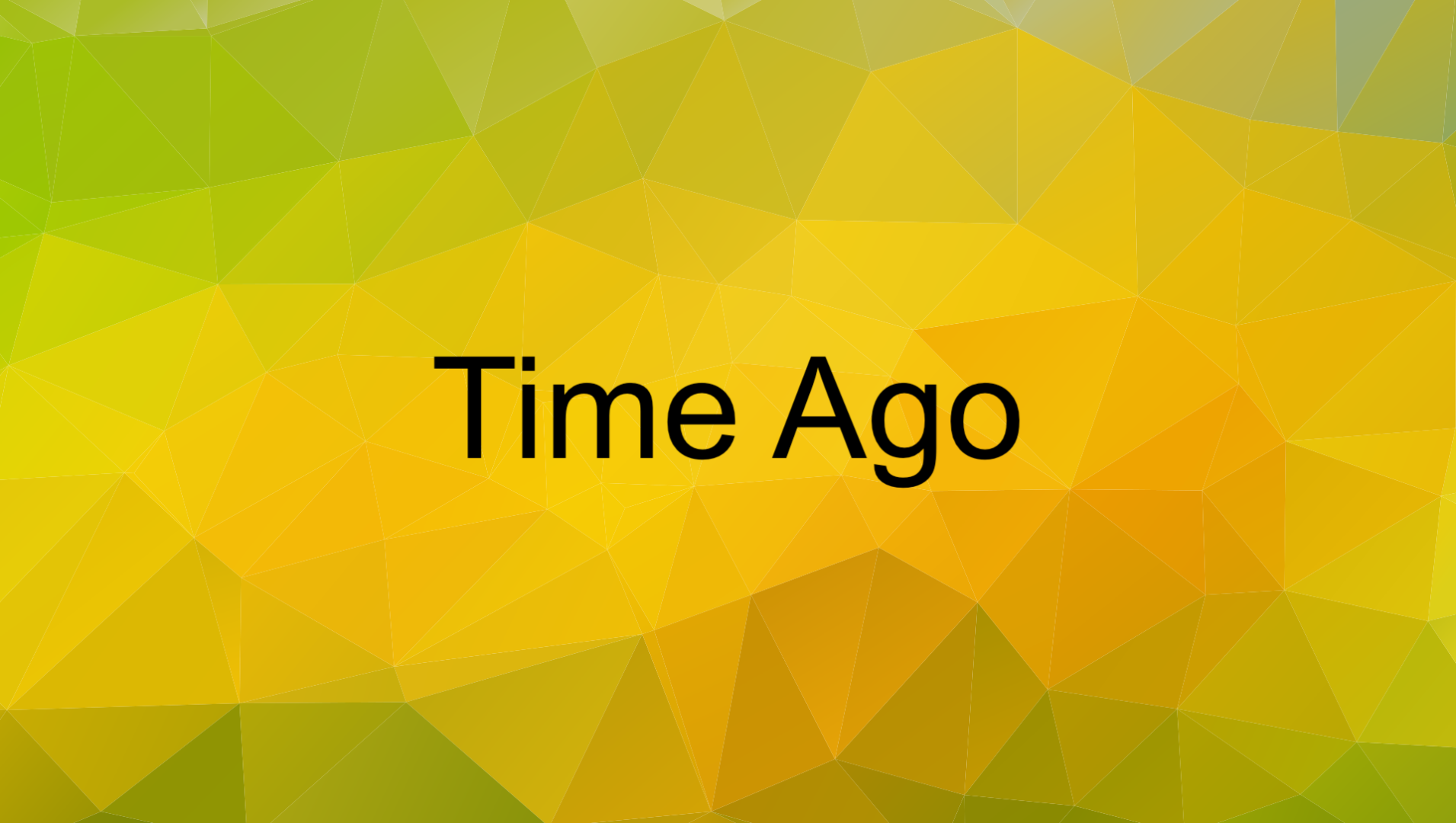
This article shows you 2 approaches to convert DateTime to time ago format in Flutter (and Dart, too), for example, 5 minutes ago, 1 day ago, 2 days ago… The first one uses the difference() method provided by the DateTime class, and the second one uses a third-party plugin.
Table of Contents
Using the difference() method
This solution is for people who want to use as few third-party plugins as possible in their projects.
Define a self-made converting function:
String convertToAgo(DateTime input){
Duration diff = DateTime.now().difference(input);
if(diff.inDays >= 1){
return '${diff.inDays} day(s) ago';
} else if(diff.inHours >= 1){
return '${diff.inHours} hour(s) ago';
} else if(diff.inMinutes >= 1){
return '${diff.inMinutes} minute(s) ago';
} else if (diff.inSeconds >= 1){
return '${diff.inSeconds} second(s) ago';
} else {
return 'just now';
}
}
Try it:
void main() {
DateTime time1 = DateTime.parse("2023-01-01 20:18:04Z");
print(convertToAgo(time1));
DateTime time2 = DateTime.utc(2022, 12, 08);
print(convertToAgo(time2));
}
The output depends on WHEN you run the code. Here is mine on January 08, 2022:
6 day(s) ago
31 day(s) ago
Note: You can improve the converting function by checking between singular and plural nouns (day/days, hour/hours, minute/minutes, second/seconds) to remove the parentheses. For example:
if(diff.inDays > 1){
return '${diff.inDays} days ago';
} else if(diff.inDays == 1){
return 'a day ago';
}
Using a third-party plugin
This solution is for people who want to write as little code as possible.
The plugin we’ll use is called timeago.
1. Add timeago and its version to the dependencies section in your pubspec.yaml file by performing the following:
dart pub add timeago
2. Run the following command:
flutter pub get
3. Import it to your code:
import 'package:timeago/timeago.dart' as timeago;
4. Use it in the code:
import 'package:timeago/timeago.dart' as timeago;
main() {
final DateTime time1 = DateTime.parse("1987-07-20 20:18:04Z");
final DateTime time2 = DateTime.utc(1989, 11, 9);
print(timeago.format(time1));
print(timeago.format(time2, locale: 'en_short'));
}
Output:
35 years ago
32 yr
Conclusion
We’ve walked through more than one technique to turn DateTime into the time ago format in Dart and Flutter. Choose the approach that suits your need. If you’d like to learn more about date-time stuff, take a look at the following articles:
- Flutter: Convert UTC Time to Local Time and Vice Versa
- 4 Ways to Format DateTime in Flutter
- How to convert String to DateTime in Flutter and Dart
- Working with Timer and Timer.periodic in Flutter
- Dart: Convert Timestamp to DateTime and vice versa
- How to implement a Date Range Picker in Flutter
You can also take a tour around our Flutter topic page and Dart topic page to see the latest tutorials and examples.